Dynamically adding rounded corners to images can be a tricky process. Making sure those corners are transparent adds an additional level of complexity.
In the project that I am working on, we used to fake the rounded corners with ImageMagick. Since our site had a white background, applying white, pre-made corners over the image was a solution.
However, a newer version of the project allows the administrator to alter the default background color for the site. I do not claim to be an ImageMagick guru, but I had worked with it before. A search in Google led me to this list of possible solutions. After looking up the commands in the thread, I had learned more, but had yet to find a solution for dynamic images. The other tutorials that I was able to find were incomplete, hard to understand (without a depth of ImageMagick knowledge), or just poorly written.
So, for your coding pleasure, I am going to show you how to create transparent, rounded corners, and provide you with the source images to do so.
For ease of understanding, I will separate the commands into individual statements. I chose to use a .jpg image with less than great quality so you can see how well the corners are applied. The source images do not necessarily match the file names in the code. In addition, this example is for PHP (hence the exec statements). The image that we are going to use is this scene from The Princess Bride (princessbride1.jpg):
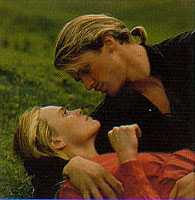
First we need to convert our image to a PNG:
$image_name = 'princess1.jpg'; exec ( "convert {$image_name} {$image_name}.png" ); |
This results in our .png image (princessbride2.png):
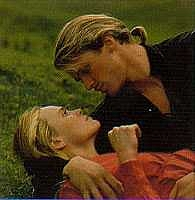
Now that our image is in a format which supports transparencies, we can go ahead and lay the colored corners upon the image. The colored corners are available for download at the end of this, but here’s an example of one of them on their own:

Here is the code that will correctly lay the corner on your image:
$image_path = '/images/'; exec( "composite -compose Over -quality 100 -gravity NorthWest $image_path/cornermask_upper_left.png {$image_name}.png {$image_name}nw " ); |
This will place our corner image over the top-left corner of the image. We want to go ahead and do this for the other corners as well making sure to use the image that was just created as the source. This will be our image name with nw appended to the end of it:
exec( "composite -compose Over -quality 100 -gravity SouthWest $image_path/cornermask_lower_left.png {$image_name}nw {$image_name}sw" ); exec( "composite -compose Over -quality 100 -gravity NorthEast $image_path/cornermask_upper_right.png {$image_name}sw {$image_name}ne" ); exec( "composite -compose Over -quality 100 -gravity SouthEast $image_path/cornermask_lower_right.png {$image_name}ne {$image_name}mask" ); |
This will result in our image with the new pink corners layed over the top. The reason that we are doing quality 100 is so that the colors don’t distort at all. This is important for the next step. Here’s our image so far (princessbride3.png):
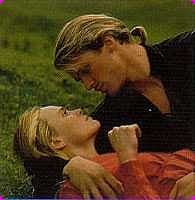
We’re just about complete. Now we need to convert those corners to transparent. The color of the corners is the hex value #ff00f6. If you want to change the colors, simply recolor the corner images and insert your own hex value in the following statement:
exec( "convert {$image_name}mask -transparent \"#ff00f6\" {$image_name}.png" ); |
That leaves us with our final image with it’s nice, new rounded corners (princessbride4.png):
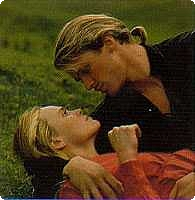
Make sure you clean up after yourself. We’ll assume you have a function called handleError() to do the error handling:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | if(!@unlink("{$image_name}nw")) { handleError(); } if(!@unlink("{$image_name}ne")) { handleError(); } if(!@unlink("{$image_name}sw")) { handleError(); } if(!@unlink("{$image_name}")) { handleError(); } if(!@unlink("{$image_name}mask")) { handleError(); } |
And there you have it. I understand there are different methods for doing this and probably a cleaner one-line version for ImageMagick. But, I hope this helps to get you on your way.
As promised, here are the corner images:




Or you can grab them in this handy .zip file: cornerimages.zip.
I hope you enjoyed this article and found it helpful. If so: please consider subscribing to my RSS feed.
Pingback: Remmrit Bookmarking()