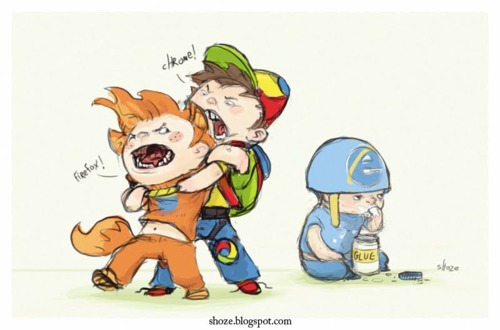
And if I had a gun, with two bullets, and I was in a room with Hitler, Bin Laden and Internet Explorer, I would shoot Internet Explorer twice.
When creating HTML elements with jQuery, there is an elegant syntax that was introduced quite a few versions ago.
$("<div/>", { id: 'someDiv', class: 'someClass', text: 'Some text in the div.' }).appendTo("#someOtherDiv"); // Another example $("<label/>", { for: 'someInput', text: 'Label for my Input' }).appendTo('#selectDialogue'); |
Simple, elegant and easy to use. This code works fine in Google Chrome and Firefox.
Unfortunately, every developers favorite browser, Internet Explorer, chokes with the following error message:
…expected identifier, string or number…
It took me a while to figure it out but, through trial and error, I discovered that the issue was with the way Internet Explorer handle reserved words. In this case, both examples are using two that IE will simply choke on. Here are the offending lines:
class: 'someClass' for: 'someInput' |
Internet Explorer will not allow you to set these attributes when creating the element. After spending too much time tracking down and figuring out the problem, since IE is so good at explaining itself when it comes to errors, I came to a solution. My first reaction was to simply apply the attribute to the element after it had been generated. After speaking with a colleague about the issue, he suggested putting the attribute name in quotes to see if it would do the trick. Turns out: this worked just fine.
After some digging around, I came across some documentation available from the jQuery folks that describes this problem. Unfortunately, this documentation was not prevalent in any of the searches I was attempting to perform. Here’s what they had to say (emphasis added):
As of jQuery 1.4, the second argument to jQuery() can accept a map consisting of a superset of the properties that can be passed to the .attr() method. Furthermore, any event type can be passed in, and the following jQuery methods can be called: val, css, html, text, data, width, height, or offset. The name “class” must be quoted in the map since it is a JavaScript reserved word, and “className” cannot be used since it is not the correct attribute name.
Using the quoted attribute solution, our code changes to:
$("<div/>", { id: 'someDiv', 'class': 'someClass', text: 'Some text in the div.' }).appendTo("#someOtherDiv"); // Another example $("<label/>", { 'for': 'someInput', text: 'Label for my Input' }).appendTo('#selectDialogue'); |
This allows Internet Explorer to go along it’s merry way doing it’s browser impression as best as it possibly can.
I hope this helps post will help others who come across this problem. If you liked this post, please consider subscribing to my feed.
Pingback: jquery ie8 append not working | bruinrow()